- /*****************************************************************
- //文件名 :1602.h
- //描述 :该头文件定义与1602有关的各种接口、函数,适用于MSP430F149
- //编写人 :小邪@清水
- //版本号 :1.00
- *****************************************************************/
- #include
- #include "1602.h"
- #define uchar unsigned char
- #define uint unsigned int
- uchar NUM[] = {"0123456789."};
- /*****************************************************************
- //关于1602的一些宏定义
- //注意:除第三个外都要根据实际使用IO口更改
- *****************************************************************/
- #define DataDir P4DIR
- #define DataPort P4OUT
- #define Busy 0x80
- #define CtrlDir P3DIR
- #define CLR_RS P3OUT&=~BIT0; //RS = P3.0
- #define SET_RS P3OUT|=BIT0;
- #define CLR_RW P3OUT&=~BIT1; //RW = P3.1
- #define SET_RW P3OUT|=BIT1;
- #define CLR_EN P3OUT&=~BIT2; //EN = P3.2
- #define SET_EN P3OUT|=BIT2;
- /*************************************************************************
- //名称 : delay
- //参数 : 无
- //返回值:无
- //功能 : 延时 5 ms的时间
- *************************************************************************/
- void Delay5ms(void)
- {
- uint i=40000;
- while (i != 0)
- {
- i--;
- }
- }
- /*************************************************************************
- //名称 :WaitForEnable
- //参数 :无
- //返回值:无
- //功能 :等待直到1602完成当前操作
- *************************************************************************/
- void WaitForEnable(void)
- {
- P4DIR &= 0x00; //将P4口切换为输入状态
- CLR_RS;
- SET_RW;
- _NOP();
- SET_EN;
- _NOP();
- _NOP();
- while((P4IN & Busy)!=0); //检测忙标志
- CLR_EN;
- P4DIR |= 0xFF; //将P4口切换为输出状态
- }
- /*************************************************************************
- //名称 :WriteCommand
- //参数 :cmd--命令,chk--是否判忙的标志,1:判忙,0:不判
- //返回值:无
- //功能 :向1602写指令
- *************************************************************************/
- void WriteCommand(uchar cmd,uchar chk)
- {
- if (chk) WaitForEnable(); // 检测忙信号
- CLR_RS;
- CLR_RW;
- _NOP();
- DataPort = cmd; //将命令字写入数据端口
- _NOP();
- SET_EN; //产生使能脉冲信号
- _NOP();
- _NOP();
- CLR_EN;
- }
- /*************************************************************************
- //名称 :WriteData
- //参数 :unsigned char Data
- //返回值:无
- //功能 :向1602写入数据
- *************************************************************************/
- void WriteData( uchar data )
- {
- WaitForEnable(); //等待液晶不忙
- SET_RS;
- CLR_RW;
- _NOP();
- DataPort = data; //将显示数据写入数据端口
- _NOP();
- SET_EN; //产生使能脉冲信号
- _NOP();
- _NOP();
- CLR_EN;
- }
- [page]
- /*************************************************************************
- //名称 :LocateXY
- //参数 :unsigned char x,unsigned char y
- //返回值:无
- //功能 :确定1602写入数据的位置,X为行坐标,Y为列坐标(都从0开始)
- *************************************************************************/
- void LocateXY(uchar x,uchar y)
- {
- uchar temp;
- temp = x&0x0f;
- y &= 0x01;
- if(y) temp |= 0x40; //如果在第2行
- temp |= 0x80;
- WriteCommand(temp,1);
- }
- /*************************************************************************
- //名称 :LcdInit
- //参数 :无
- //返回值:无
- //功能 :1602初始化
- *************************************************************************/
- void LcdInit(void)
- {
- CtrlDir |= 0x07; //控制线端口设为输出状态
- DataDir |= 0xFF; //数据端口设为输出状态
- WriteCommand(0x38, 0); //规定的复位操作
- Delay5ms();
- WriteCommand(0x38, 0);
- Delay5ms();
- WriteCommand(0x38, 0);
- Delay5ms();
- WriteCommand(0x38, 1); //显示模式设置
- WriteCommand(0x08, 1); //显示关闭
- WriteCommand(0x01, 1); //显示清屏
- WriteCommand(0x06, 1); //写字符时整体不移动
- WriteCommand(0x0c, 1); //显示开,不开游标,不闪烁
- }
- /*************************************************************************
- //名称 :WriteStr
- //参数 :待写入数组的首地址,unsigned int n,unsigned char x,unsigned char y
- //返回值:无
- //功能 :在给定位置显示一个数组,长度为l
- *************************************************************************/
- void WriteStr(uchar *a,uint l,uchar x,uchar y)
- {
- uchar i;
- LocateXY(x,y);
- for(i = 0;i < l;i ++)
- WriteData(a[i]);
- }
- /*************************************************************************
- //名称 :WriteNum
- //参数 :待写入数字,unsigned char x,unsigned char y
- //返回值:无
- //功能 :在给定位置显示一个数字(不超过5位且小于65536)
- *************************************************************************/
- void WriteNum(uint n,uchar x,uchar y)
- {
- uchar five,four,three,two,one;
- LocateXY(x,y);
- if((n >= 10000)&&(n <= 65535))
- {
- five = n / 10000;
- four = (n % 10000) / 1000;
- three = ((n - five * 10000) % 1000) / 100;
- two = ((n - five * 10000) % 1000 - three * 100 ) / 10;
- one = ((n - five * 10000) % 1000 - three * 100 ) % 10;
- WriteData(NUM[five]);
- WriteData(NUM[four]);
- WriteData(NUM[three]);
- WriteData(NUM[two]);
- WriteData(NUM[one]);
- }
- if((n >= 1000)&&(n <= 9999))
- {
- four = n / 1000;
- three = (n % 1000) / 100;
- two = (n % 1000 - three * 100 ) / 10;
- one = (n % 1000 - three * 100 ) % 10;
- WriteData(NUM[four]);
- WriteData(NUM[three]);
- WriteData(NUM[two]);
- WriteData(NUM[one]);
- }
- if((n >= 100)&&(n <= 999))
- {
- three = n / 100;
- two = (n - three * 100 ) / 10;
- one = (n - three * 100 ) % 10;
- WriteData(NUM[three]);
- WriteData(NUM[two]);
- WriteData(NUM[one]);
- }
- if((n >= 10)&&(n <= 99))
- {
- two = n / 10;
- one = n % 10;
- WriteData(NUM[two]);
- WriteData(NUM[one]);
- }
- if((n > 0)&&(n <= 9))WriteData(NUM[n]);
- }
- /*************************************************************************
- //名称 :WriteFloat
- //参数 :待写入浮点数,unsigned char x,unsigned char y
- //返回值:无
- //功能 :在给定位置显示一个浮点数(整数部分和小数部分都不超过两位)
- *************************************************************************/
- void WriteFloat(float n,uchar x,uchar y)
- {
- uint Integer,Decimal; //Integer用于存放整数部分,Decimal用于存放小数部分
- Integer = (uint)(n/1);
- Decimal = (uint)(n * 100 - Integer * 100);
- WriteNum(Integer,x,y);
- WriteData(NUM[10]);
- WriteNum(Decimal,x+3,y);
- }
上一篇:12864控制forMSP430
下一篇:矩阵键盘控制forMSP430
推荐阅读最新更新时间:2024-03-16 14:32
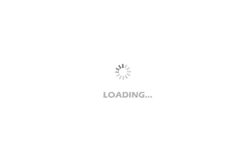
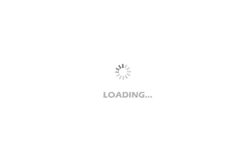
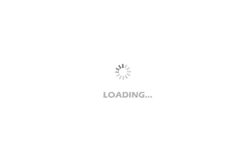
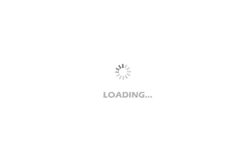
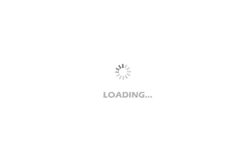