前几天写一个东西,要用到STM32F103的串口1和串口2以不同的波特率交换数据,也就是说串口1波特率为9600,串口2波特率为115200,串口1可以把接收到的数据通过串口2发送出去,串口2也可以把接收到的数据通过串口1发送出去。低波特率向高波特率发送数据没问题,高波特率向低波特率发送数据会丢数据,原因是低波特率的串口还没发送完数据高波特率的串口就又发数据过来了,处理不过来。在同事的在帮助下,写出一个先进先出环形队列(FIFO)程序。接收数据用中断,发送数据用在主函数中查询发送完成标志位。希望对大家有点帮助,可能程序不完美,但程序可以用。定义一个fifo.h部件和一个fifo.c文件。其他的都在主函数中调用。
#ifndef _FIFO_H_
#define _FIFO_H_
#include "stm32f10x.h"
#define FIFO_SIZE 256
typedef struct
{
uint8_t Buf[FIFO_SIZE]; //定义缓冲区,大小用FIFO_SIZE定义
volatile uint32_t Read; //定义读取指针
volatile uint32_t Write; //定义写入指针
}FIFOTypeDef;
extern FIFOTypeDef USART1_fifo;
extern FIFOTypeDef USART2_fifo;
void PutChar(uint8_t c);
uint8_t GetChar(uint8_t *c);
void PutChar1(uint8_t c);
uint8_t GetChar1(uint8_t *c);
#endif
#include "fifo.h"
FIFOTypeDef USART1_fifo; //定义串口1的队列fifo
FIFOTypeDef USART2_fifo; //定义串口2的队列fifo
/*******************************************************************************
* Function Name : PutChar
* Description : put a fifo value into fifo buffer
* Input : c
* Output : None
* Return : None
*******************************************************************************/
void PutChar(uint8_t c)
{
USART1_fifo.Buf[USART1_fifo.Write] = c; //写入数据到缓冲区
if(++USART1_fifo.Write >= FIFO_SIZE) //写入指针超出缓冲区最大值,返回最初位置
{
USART1_fifo.Write = 0;
}
}
/*******************************************************************************
* Function Name : GetChar
* Description : read fifo value from fifo buffer with timeout feature
* Input : c
* Output : None
* Return : 0
*******************************************************************************/
uint8_t GetChar(uint8_t *c)
{
if(USART1_fifo.Read == USART1_fifo.Write) //如果没有存入数据,则返回0
{
return 0;
}
else
{
*c = USART1_fifo.Buf[USART1_fifo.Read]; //读取数据,传入到指针c
if (++USART1_fifo.Read >= FIFO_SIZE) //读取指针超出缓冲区最大值,返回最初位置
{
USART1_fifo.Read = 0;
}
return 1; //成功读取数据返回1
}
}
/*******************************************************************************
* Function Name : PutChar
* Description : put a fifo value into fifo buffer
* Input : c
* Output : None
* Return : None
*******************************************************************************/
void PutChar1(uint8_t c)
{
USART2_fifo.Buf[USART2_fifo.Write] = c;
if(++USART2_fifo.Write >= FIFO_SIZE)
{
USART2_fifo.Write = 0;
}
}
/*******************************************************************************
* Function Name : GetChar
* Description : read fifo value from fifo buffer with timeout feature
* Input : c
* Output : None
* Return : 0
*******************************************************************************/
uint8_t GetChar1(uint8_t *c)
{
if(USART2_fifo.Read == USART2_fifo.Write)
{
return 0;
}
else
{
*c = USART2_fifo.Buf[USART2_fifo.Read];
if (++USART2_fifo.Read >= FIFO_SIZE)
{
USART2_fifo.Read = 0;
}
return 1;
}
}
#include "stm32f10x.h"
#include "fifo.h"
/*******************************************************************************
* Function Name : UIF_Init
* Description : 初始化串口
* Input : USARTx = USART1,2,3
* : BPS = 1200, 4800,,,
* : STB = 1,5,2,15
* Return : None
*******************************************************************************/
void UIF_Init(USART_TypeDef* USARTx, uint32_t BPS, uint32_t STB)
{
uint32_t Dly;
USART_InitTypeDef USART_InitStruct; //定义串口结构体
NVIC_InitTypeDef NVIC_InitStructure; //定义中断结构体
USART_ClearFlag(USARTx, USART_FLAG_TC);
USART_DeInit(USARTx); //复位串口x
USART_InitStruct.USART_BaudRate = BPS;
USART_InitStruct.USART_WordLength = USART_WordLength_8b; //8位字节长度数据
if(STB == 1)
USART_InitStruct.USART_StopBits = USART_StopBits_1;
else if(STB == 5)
USART_InitStruct.USART_StopBits = USART_StopBits_0_5;
else if(STB == 15)
USART_InitStruct.USART_StopBits = USART_StopBits_1_5;
else
USART_InitStruct.USART_StopBits = USART_StopBits_2;
USART_InitStruct.USART_Parity = USART_Parity_No; //无奇偶检验位
USART_InitStruct.USART_HardwareFlowControl = USART_HardwareFlowControl_None;//无硬件数据流控制
USART_InitStruct.USART_Mode = USART_Mode_Rx | USART_Mode_Tx; //接收 发送模式
USART_Init(USARTx, &USART_InitStruct); //初始化串口x
NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn; //使能串口1中断
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 3; //先占优先级2级
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0; //从优先级2级
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; //使能外部中断通道
NVIC_Init(&NVIC_InitStructure); //根据NVIC_InitStruct中指定的参数初始化外设NVIC寄存器
NVIC_InitStructure.NVIC_IRQChannel = USART2_IRQn; //使能串口2中断
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 3; //先占优先级2级
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 1; //从优先级2级
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; //使能外部中断通道
NVIC_Init(&NVIC_InitStructure); //根据NVIC_InitStruct中指定的参数初始化外设NVIC寄存器
if(USART_GetFlagStatus(USARTx, USART_FLAG_TC)==SET)
USART_ClearFlag(USARTx, USART_FLAG_TC);
USART_Cmd(USARTx, ENABLE); //使能串口x
for(Dly = 0; Dly < 0xFFFF; Dly++);
USART_ClearFlag(USARTx, USART_FLAG_TC);
USART_ITConfig(USARTx,USART_IT_RXNE,ENABLE); //开启接收中断
}
/*******************************************************************************
* Function Name : GPIO_Config
* Description : 初始化IO
* Input : None
* Return : None
*******************************************************************************/
void GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA
| RCC_APB2Periph_GPIOB
| RCC_APB2Periph_AFIO
| RCC_APB2Periph_USART1, ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_9; //USART1_TX Output
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(GPIOA, &GPIO_InitStruct);
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_10; //USART1_RX Input
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStruct);
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_2; //USART2_TX Output
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(GPIOA, &GPIO_InitStruct);
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_3; //USART2_RX Input
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStruct);
}
/*******************************************************************************
* Function Name : NVIC_Configuration
* Description : 设置NVIC中断分组
* Input : None
* Return : None
*******************************************************************************/
void NVIC_Configuration(void)
{
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2); //设置NVIC中断分组2:2位抢占优先级,2位响应优先级
}
/*******************************************************************************
* Function Name : USART_SendByte
* Description : 发送一个字节
* Input : USARTx = USART1,2,3
Data = 要发送的数据
* Return : None
*******************************************************************************/
void USART_SendByte(USART_TypeDef* USARTx, uint16_t Data)
{
USARTx->DR = (Data & (uint16_t)0x01FF);;
while(USART_GetFlagStatus(USARTx, USART_FLAG_TC) != SET);
}
/*******************************************************************************
* Function Name : main
* Description : 主函数
* Input : None
* Return : None
*******************************************************************************/
int main(void)
{
uint8_t byte;
GPIO_Config();
NVIC_Configuration();
UIF_Init(USART1,115200,1); //串口1波特率115200,1位停止位
UIF_Init(USART2,9600,1); //串口2波特主9600,1位停止位 //使能串口
while(1)
{
if(USART1_fifo.Write != USART1_fifo.Read) //如果读取指针不等于写入指针,说明缓冲区有数据
{
if(GetChar(&byte)) //如果读取数据成功,返回1
{
USART_SendByte(USART2,byte); //把串口1的缓冲区数据通过串口2发送出去
}
}
if(USART2_fifo.Read!=USART2_fifo.Write) //如果读取指针不等于写入指针,说明缓冲区有数据
{
if(GetChar1(&byte)) //如果读取数据成功,返回1
{
USART_SendByte(USART1,byte); //把串口2的缓冲区数据通过串口1发送出去
}
}
}
}
/*******************************************************************************
* Function Name : USART1_IRQHandler
* Description : 串口1中断服务程序
* Input : None
* Return : None
*******************************************************************************/
void USART1_IRQHandler(void)
{
u8 res;
if(USART_GetITStatus(USART1, USART_IT_RXNE) != RESET) //如果接收到数据
{
USART_ClearITPendingBit(USART1,USART_IT_RXNE);//清空接收标志
res = USART_ReceiveData(USART1);
PutChar(res); //压入数据到串口1缓冲区
}
//溢出,如果发生溢出需要先读SR,再读DR寄存器则可清除不断入中断的问题
if(USART_GetFlagStatus(USART1,USART_FLAG_ORE)==SET)
{
USART_ClearFlag(USART1,USART_FLAG_ORE); //读SR其实就是清除标志
USART_ReceiveData(USART1); //读DR
}
}
/*******************************************************************************
* Function Name : USART2_IRQHandler
* Description : 串口2中断服务程序
* Input : None
* Return : None
*******************************************************************************/
void USART2_IRQHandler(void)
{
u8 Res;
if(USART_GetITStatus(USART2, USART_IT_RXNE) != RESET) //接收中断
{
USART_ClearITPendingBit(USART2,USART_IT_RXNE);//清空接收标志
Res =USART_ReceiveData(USART2);//(USART2->DR); //读取接收到的数据
PutChar1(Res); //压入数据到串口2缓冲区
}
//溢出,如果发生溢出需要先读SR,再读DR寄存器则可清除不断入中断的问题
if(USART_GetFlagStatus(USART2,USART_FLAG_ORE)==SET)
{
USART_ClearFlag(USART2,USART_FLAG_ORE); //读SR其实就是清除标志
USART_ReceiveData(USART2); //读DR
}
}
上一篇:STM32 串口详细讲解
下一篇:STM32F103 SPI3总结
推荐阅读最新更新时间:2024-03-16 16:27
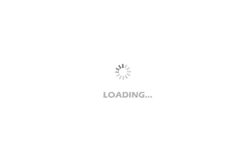
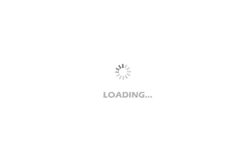
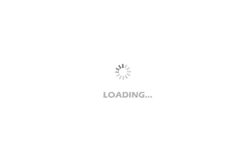
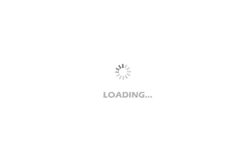