12684液晶屏,bmp180传感器,51单片机
单片机源程序如下:
#include #include #include #include #include #include #include "lcd.h" #include "BMP180.H" /*定义传感器内部的EEPROM存储单元里面11个值*/ #define uchar unsigned char #define uint unsigned int typedef unsigned char BYTE; typedef unsigned short WORD; #define BMP085_SlaveAddress 0xee //定义器件在IIC总线中的从地址 short ac1; short ac2; short ac3; unsigned short ac4; unsigned short ac5; unsigned short ac6; short b1; short b2; uchar idata flag; int idata AltitudeTemp[10]={0,0,0,0,0,0,0,0,0,0}; // 海拔高度的10个临时值,取平均值 short mb; short mc; short md; #define OSS 0// Oversampling Setting (note: code is not set up to use other OSS values) uchar code PressureTitle[16]= {"气压温度测量"}; uchar idata ShowPressure[16]= {"高度: 米"}; uchar idata ShowTemperature[16]={"温度: . ℃"}; uchar idata ShowAltitude[16]= {"电压: . V"}; uchar code HZW0[10]={"欢迎使用"}; uchar code HZW1[12]={"海拔高度计"}; uchar code Blank[16]; bit ack; sbit DA = P2^0; sbit CK = P2^1; sbit CS = P2^2; uchar TaskFlag; //*延时us级函数 void Delay5us() { _nop_();_nop_();_nop_();_nop_(); _nop_();_nop_();_nop_();_nop_(); _nop_();_nop_();_nop_();_nop_(); _nop_();_nop_();_nop_();_nop_(); } /************************************** 延时5毫秒(STC90C52RC@12M) 不同的工作环境,需要调整此函数 当改用1T的MCU时,请调整此延时函数 **************************************/ void Delay1ms(uint xms) { uint a,b; for(a=0;a<110;a++) for(b=0;b void Delay5ms() { WORD n = 560; while (n--); } /************************************** 起始信号 **************************************/ void BMP085_Start() { SDA = 1; //拉高数据线 SCL = 1; //拉高时钟线 Delay5us(); //延时 SDA = 0; //产生下降沿 Delay5us(); //延时 SCL = 0; //拉低时钟线 } /************************************** 停止信号 **************************************/ void BMP085_Stop() { SDA = 0; //拉低数据线 SCL = 1; //拉高时钟线 Delay5us(); //延时 SDA = 1; //产生上升沿 Delay5us(); //延时 } /************************************** 发送应答信号 入口参数:ack (0:ACK 1:NAK) **************************************/ void BMP085_SendACK(bit ack) { SDA = ack; //写应答信号 SCL = 1; //拉高时钟线 Delay5us(); //延时 SCL = 0; //拉低时钟线 Delay5us(); //延时 } /************************************** 接收应答信号 **************************************/ bit BMP085_RecvACK() { SCL = 1; //拉高时钟线 Delay5us(); //延时 CY = SDA; //读应答信号 SCL = 0; //拉低时钟线 Delay5us(); //延时 return CY; } /************************************** 向IIC总线发送一个字节数据 **************************************/ void BMP085_SendByte(BYTE dat) { BYTE i; for (i=0; i<8; i++) //8位计数器 { dat <<= 1; //移出数据的最高位 SDA = CY; //送数据口 SCL = 1; //拉高时钟线 Delay5us(); //延时 SCL = 0; //拉低时钟线 Delay5us(); //延时 } BMP085_RecvACK(); } /************************************** 从IIC总线接收一个字节数据 **************************************/ BYTE BMP085_RecvByte() { BYTE i; BYTE dat = 0; SDA = 1; //使能内部上拉,准备读取数据, for (i=0; i<8; i++) //8位计数器 { dat <<= 1; SCL = 1; //拉高时钟线 Delay5us(); //延时 dat |= SDA; //读数据 SCL = 0; //拉低时钟线 Delay5us(); //延时 } return dat; } //********************************************************* //读出BMP085内部数据,连续两个 //********************************************************* short Multiple_read(uchar ST_Address) { uchar msb, lsb; short _data; BMP085_Start(); //起始信号 BMP085_SendByte(BMP085_SlaveAddress); //发送设备地址+写信号 BMP085_SendByte(ST_Address); //发送存储单元地址 BMP085_Start(); //起始信号 BMP085_SendByte(BMP085_SlaveAddress+1); //发送设备地址+读信号 msb = BMP085_RecvByte(); //BUF[0]存储 BMP085_SendACK(0); //回应ACK lsb = BMP085_RecvByte(); BMP085_SendACK(1); //最后一个数据需要回NOACK BMP085_Stop(); //停止信号 Delay5ms(); _data = msb << 8; _data |= lsb; return _data; } //******************************************************************** long bmp085ReadTemp(void) { BMP085_Start(); //起始信号 BMP085_SendByte(BMP085_SlaveAddress); //发送设备地址+写信号 BMP085_SendByte(0xF4); // write register address BMP085_SendByte(0x2E); // write register data for temp BMP085_Stop(); //发送停止信号 Delay1ms(10);// max time is 4.5ms return (long) Multiple_read(0xF6); } //************************************************************* long bmp085ReadPressure(void) { long pressure = 0; BMP085_Start(); //起始信号 BMP085_SendByte(BMP085_SlaveAddress); //发送设备地址+写信号 BMP085_SendByte(0xF4); // write register address BMP085_SendByte(0x34); // write register data for pressure BMP085_Stop(); //发送停止信号 Delay1ms(10); // max time is 4.5ms pressure = Multiple_read(0xF6); pressure &= 0x0000FFFF; return pressure; } //************************************************************** //初始化BMP085,根据需要请参考pdf进行修改************** void Init_BMP085() { ac1 = Multiple_read(0xAA); ac2 = Multiple_read(0xAC); ac3 = Multiple_read(0xAE); ac4 = Multiple_read(0xB0); ac5 = Multiple_read(0xB2); ac6 = Multiple_read(0xB4); b1 = Multiple_read(0xB6); b2 = Multiple_read(0xB8); mb = Multiple_read(0xBA); mc = Multiple_read(0xBC); md = Multiple_read(0xBE); } void da5615(unsigned int da) { unsigned char i; da <<= 6;//10有效数据左对齐 CS = 0; CK = 0; for (i=0;i<12;i++) { DA = (bit)(da & 0x8000); CK = 1;
上一篇:单片机+MAX6675热电偶(热电堆)智能体温检测系统
下一篇:超详细的单片机交通信号灯控制程序
推荐阅读最新更新时间:2024-11-07 06:44
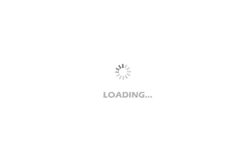