基于stm8的ds1302模块千万别把数据写在第3个区,不然卡就锁住了,只能写在第2个区
电路原理图如下:
stm8单片机源码:
/* Includes ------------------------------------------------------------------*/
/* Includes ------------------------------------------------------------------*/
#include "stm8s.h"
#include "stm8s_clk.h"
#include "intrinsics.h"
#include "stm8s_uart1.h"
#include "uart.h"
#include "rc522.h"
#include "string.h"
#include "IWDG.h"
#include "tim2.h"
#include "1602i2c.h"
void Delay(u32 nCount);
extern u8 RxBuffer[RxBufferSize];
extern u8 UART_RX_NUM;
extern int time2;
extern int tim2test;
extern int tim2test1;
unsigned char CT[2];//卡类型
unsigned char SN[4]; //卡号
unsigned char M1UID[8]={0x2e,0x55,0,0,0,0,0,0};//读卡器向上发送,读到卡后自动发
extern unsigned char controlflag;//接收到控制LED beep指令标志 01表示收到
extern unsigned char sendflag;//发送标志 =1表示还未发送过 =0表示已经发送一次 等待回复 如果超时重新发送
unsigned char write[16] = {0x01,0x02,0x03,0x04,0x05,0x06,0x07,0x08,0x09,0x0A,0x0B,0x0C,0x0D,0x0E,0x0F,0x10};
unsigned char read[16] = {0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00};
extern unsigned char receive[8];
u8 read_flag=0;//读卡标志位 1;读到卡 0;还没读到卡
u8 time_flag=0;//超时标志 0;未超时 1:超时
//unsigned char key[6] = {0x19,0x84,0x07,0x15,0x76,0x14};
unsigned char key[6] = {0xff,0xff,0xff,0xff,0xff,0xff};
int sum=0;
//unsigned char key[6] = {0x00,0x00,0x00,0x00,0x00,0x00};
/* Private macro -------------------------------------------------------------*/
#define countof(a) (sizeof(a) / sizeof(*(a)))
#define BufferSize (countof(Tx_Buffer)-1)
/* Private variables ---------------------------------------------------------*/
u8 Tx_Buffer[] = "请刷卡";
u8 Rx_Buffer[BufferSize];
u32 FLASH_ID ;
/* Private defines -----------------------------------------------------------*/
/* Private function prototypes -----------------------------------------------*/
void cardNo2String(u8 *cardNo, u8 *str);
/* Private functions ---------------------------------------------------------*/
#define ReadWriteAddr 3 //读写扇区
void SN_UID(void)
{
unsigned int tempUID;
M1UID[2]=SN[0];
M1UID[3]=SN[1];
M1UID[4]=SN[2];
M1UID[5]=SN[3];
tempUID=M1UID[1]+M1UID[2]+M1UID[3]+M1UID[4]+M1UID[5];
M1UID[6]=tempUID&0x00ff;
M1UID[7]=tempUID>>8;
}
void rxfromPIC(void)
{
u8 i;
sum=receive[1]+receive[2]+receive[3]+receive[4]+receive[5];
if((receive[0]==0x7e)&&(receive[1]==0x55)&&(receive[6]==(sum&0x00ff))&&(receive[7]==(sum&0xff00)>>8))
{
read_flag=0;
if(receive[2]==0x01)
{
}
else if(receive[2]!=0x01)
{
}
if(receive[3]==0x01)
{
}
else if(receive[3]!=0x01)
{
}
if(receive[4]==0x01)
{
}
receive[0]=0x7e;
UART1_SendString(receive, 8);
for(i=2;i<8;i++)
{
M1UID[i]=0;
}
tim2test1=0;//启动计时
}
for(i=0;i<8;i++)
{
receive[i]=0;
}
if(tim2test1>5000)//超时全部关闭
{
tim2test1=0;
// GPIO_HIGH(GPIOD, GPIO_PIN_3);
// GPIO_HIGH(GPIOD, GPIO_PIN_7);
// GPIO_LOW(GPIOD, GPIO_PIN_2);
}
}
void main(void)
{
unsigned char status;
/*设置内部时钟16M为主时钟*/
CLK_HSIPrescalerConfig(CLK_PRESCALER_HSIDIV2);
status = memcmp(read,write,16);//清0
InitRc522();//rc522初始化
sendflag=1;
I2C1602_Configuration();
init1602_i2c();
__enable_interrupt();//开启总中断
//////////////
write[0] =1;//
write[1] =3;//
write[2] =2;//
write[3] =6;//
write[4] =7;//
write[5] =7;//
write[6] =6;//
write[7] =0;//
write[8] =2;//
write[9] =8;//
write[10] =5;//
///////////////// 要写入的值,最多16个///////
while(1)
{
status = PcdRequest(PICC_REQALL,CT); // 扫描卡
status= PcdAnticoll(SN);
//防冲撞
rxfromPIC();
if (status==MI_OK)
{
SN_UID();
status = PcdSelect(SN); //选择要操作的卡
if (status==MI_OK)
{
if( MI_OK == PcdAuthState(0x61,ReadWriteAddr,key,SN) ) //验证A密码
{
// status = PcdWrite(2,write);//写入M1卡第2块块数据
status = PcdRead(2,read);//读取M1卡第2块块数据
if(MI_OK == status)
{
zl_i2c(0x80);
sj_i2c(0x30+ read[0]);
sj_i2c(0x30+ read[1]);
sj_i2c(0x30+ read[2]);
sj_i2c(0x30+ read[3]);
sj_i2c(0x30+ read[4]);
sj_i2c(0x30+ read[5]);
sj_i2c(0x30+ read[6]);
sj_i2c(0x30+ read[7]);
sj_i2c(0x30+ read[8]);
sj_i2c(0x30+ read[9]);
sj_i2c(0x30+ read[10]);
}
}
}
}
}
}
void Delay(u32 nCount)
{
/* Decrement nCount value */
while (nCount != 0)
{
nCount--;
}
}
void Hex2String(u8 hex,u8 *str)
{
str[0] = (hex / 100) + '0';
str[1] = (hex % 100 / 10) + '0';
str[2] = (hex % 10) + '0';
}
void cardNo2String(u8 *cardNo, u8 *str)
{
u8 Count = 0;
for(Count = 0; Count < 4; Count++)
{
Hex2String(cardNo[Count], str + Count * 4);
if(Count == 3)
{
str[15] = 'n';
}
else
{
str[Count * 4 + 3] = ':';
}
……………………
上一篇:stm8 唯一ID号加密方法.思路
下一篇:STM8L151G sx1276-LoRa测试程序
推荐阅读最新更新时间:2024-11-11 21:20
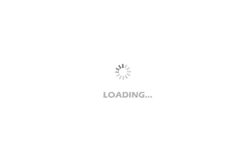
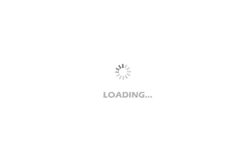
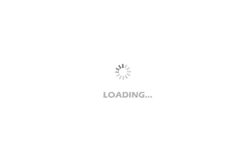
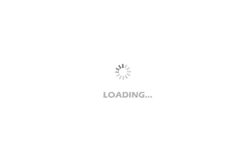
设计资源 培训 开发板 精华推荐
- LTC2910 的典型应用 - 八路正/负电压监视器
- C8051F996DK,用于工业应用的 C8051F996 8051 微控制器的开发系统
- OP484FSZ-REEL 高端负载电流监控器的典型应用
- STM32 MCU 电机控制开发系统
- LTC2946HMS 宽范围 -4V 至 -500V 负电源、电荷和能量监视器(10kHz I2C 接口)的典型应用
- #第七届立创电赛#USB电压电流表
- LT1021DCN8-10 具有升压输出电流和电流限制的电压基准的典型应用
- elrs2.4G pp接收机——ExpressLRS开源接收机
- 完整的 VCA 为视频/成像提供高达 20dB 的视频放大器
- 用于 24V 汽车应用的 LTC4367HMS8 过压电源保护控制器的典型应用