Today I accomplish a application on PIC32MZ EC Starter Kit. The feature of application is to light up LED when key released and put out LED when key pressed. LED is the Starter Kit LED1 connecting to RH0. Key is the Starter Kit push button SW1 connecting to RB12. I also use the Timer1 Interrupt. In my application, I just design the software with three modules -- LED module, KEY module and TIMER1 module and the final is the main function and interrupt service routine.
LED module let LED1 on when "LedState" variable is 1, and off when "LedState" variable is 0. Below is its implementation.
#define LED_IOCTL() TRISHCLR = (1<<0)
#define LED_SETON() LATHSET = (1<<0)
#define LED_SETOFF() LATHCLR = (1<<0)
#define LED_ONOFF() LATHINV = (1<<0)
#define LED_OPEN() ANSELH &= 0xFFFFFFFE
typedef enum _LED_STATE_t
{
OFF = 0,
ON = 1
} LED_STATE_t;
LED_STATE_t PreLedState, LedState;
void Led_Init(void)
{
LED_OPEN();
LED_IOCTL();
LED_SETON();
LedState = ON;
PreLedState = LedState;
}
void Led_Scheduler(void)
{
if (LedState != PreLedState)
{
LED_ONOFF();
PreLedState = LedState;
}
}
The "LedState" variable is determined by KEY module. The key press validated, the "LedState" is 1. The key release validated, the "LedState" is 0. Since the key (push button) does not have any debounce circuitry. I enable the internal resistor pull-up and use a debounce algorithm to remove random or spurious transistings of a digital signal read as an input by PIC32MZ. The following example illustrates how this algorithm works. The sequence labeled, corrupted, has significant random transitions added to the real signal. The sequence labled, integrator, represents the algorithm integrator which is constrained to be between 0 and 3. The sequence labeled, output, only makes a transition when the integrator reaches either 0 or 3. Note that the output signal lags the input signal by the integration time but is free of spurious transitions.
real signal 0000111111110000000111111100000000011111111110000000000111111100000
corrupted 0100111011011001000011011010001001011100101111000100010111011100010
integrator 0100123233233212100012123232101001012321212333210100010123233321010
output 0000001111111111100000001111100000000111111111110000000001111111000
The algotithm has been around for many many years but does not seem to be widely known. It is notable that the algotithm uses integration as opposed to edge logic. It is the integration that makes this algotithm so robust in the presence of noise. In the implementation of KEY module, I use "DEBOUNCE_TimeFlag" variable to control debounce start.
#define DEBOUNCE_Input (PORTB & 0x1000)
#define DEBOUNCE_Open() ANSELB = 0xFFFFEFFF
#define DEBOUNCE_IOCtl() CNPUBSET = 0x1000
#define DEBOUNCE_Output LedState
#define DEBOUNCE_ThreholdLow 0
#define DEBOUNCE_ThreholdHigh 100
unsigned long DEBOUNCE_PreInput;
unsigned char DEBOUNCE_EventStart;
unsigned int DEBOUNCE_Integrator;
volatile unsigned char DEBOUNCE_TimeFlag;
void Key_Init(void)
{
DEBOUNCE_EventStart = 0;
DEBOUNCE_Integrator = DEBOUNCE_ThreholdHigh / 2;
DEBOUNCE_TimeFlag = 0;
DEBOUNCE_Open();
DEBOUNCE_IOCtl();
DEBOUNCE_PreInput = DEBOUNCE_Input;
}
void Key_Scheduler(void)
{
if (DEBOUNCE_TimeFlag)
{
if (DEBOUNCE_EventStart)
{
if (DEBOUNCE_Input == 0)
{
if (DEBOUNCE_Integrator-- == DEBOUNCE_ThreholdLow)
{
DEBOUNCE_Output = 0;
DEBOUNCE_PreInput = DEBOUNCE_Input;
DEBOUNCE_EventStart = 0;
DEBOUNCE_Integrator = DEBOUNCE_ThreholdHigh / 2;
}
}
else //if (DEBOUNCE_Input == 1)
{
if (DEBOUNCE_Integrator++ == DEBOUNCE_ThreholdHigh)
{
DEBOUNCE_Output = 1;
DEBOUNCE_PreInput = DEBOUNCE_Input;
DEBOUNCE_EventStart = 0;
DEBOUNCE_Integrator = DEBOUNCE_ThreholdHigh / 2;
}
}
}
else if (DEBOUNCE_PreInput != DEBOUNCE_Input)
{
DEBOUNCE_EventStart = 1;
}
DEBOUNCE_TimeFlag = 0;
}
}
TIMER module uses timer1 to generate interrupt per millisecond. and set "DEBOUNCE_TimeFlag" logic 1 in the timer1 interrupt service routine.
void Timer1_Init(void)
{
T1CON = 0x8010;
PR1 = 0x30D3;
IPC1SET = 0x5;
TMR1 = 0;
IEC0SET = 0x10;
IFS0CLR = 0x10;
}
void Timer1_Write(unsigned int value)
{
TMR1 = value & 0xFFFF;
}
unsigned int Timer1_Read(void)
{
return (TMR1 & 0xFFFF);
}
Since interrupt will be used, the following sentence is enable interrupt with multi-vector mode.
#define Mvec_Interrupt() INTCONSET = 0x1000; asm volatile("ei")
The final is the implementation of main function and interrupt service and routine.
#include
#include "Led.h"
#include "Key.h"
#include "Timer.h"
#include "Interrupt.h"
#include
#include "ConfigurationBits.h"
void __ISR(_TIMER_1_VECTOR,ipl1AUTO) Timer1_Handler(void)
{
DEBOUNCE_TimeFlag = 1;
Timer1_Write(0);
IFS0CLR = 0x10; // Clear flag
}
void main(void)
{
Led_Init();
Key_Init();
Timer1_Init();
Mvec_Interrupt();
while (1)
{
Key_Scheduler();
Led_Scheduler();
}
}
The coding thing is done so far. we only need to compile and build it, then download it to PIC32MZ EC Starter Kit. You will get SW1 presse or release followed LED1 off or on. I bet it would run perfectly since the wonderful debounce algorithm.
上一篇:PIC32MZ tutorial -- Change Notification
下一篇:PIC32MZ tutorial -- Timer Interrupt
推荐阅读最新更新时间:2024-03-16 15:31
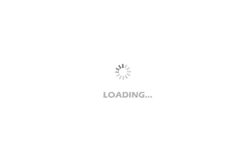
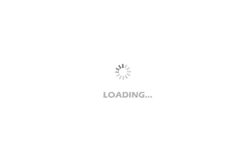
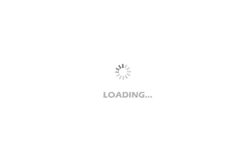
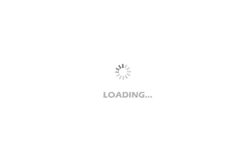
- 热门资源推荐
- 热门放大器推荐
-
Intelligent program synthesis framework and key scientific problems for embedded software
-
Microelectronic Circuits Analysis and Design 2th edition_Muhammad.H..Rashid_Cengage 2011
-
嵌入式LINUX基础教程 第2版 ((美)克里斯托弗·哈利南(Christopher Hallinan))
-
5G and E-Band Communication Circuits in Deep-Scaled CMOS
-
控制系统计算机辅助设计 — MATLAB语言与应用
-
东芝1200V SIC SBD “TRSxxx120Hx系列” 助力工业电源设备高效
-
晶体振荡器设计与温度补偿
-
ADS信号完整性仿真与实战