#include
#include
#include
#define TURE 1
#define FALSE 0
#define delayus() _nop_();_nop_();_nop_();_nop_();_nop_();_nop_();_nop_();_nop_();_nop_();_nop_();_nop_();_nop_();_nop_();_nop_();_nop_();_nop_();_nop_();_nop_();_nop_();_nop_()
/***************************************
**自定义数据类型
***************************************/
typedef unsigned char uint8;
typedef unsigned int uint16;
typedef unsigned long uint32;
/***************************************
**按键消息结构体
***************************************/
typedef struct
{
uint8 handle; //功能索引
uint8 assignments; //键值
uint8 time; //键按下的时间
} K_MSG;
/******************************************
** 端口定义
******************************************/
sbit LCD_E = P2^7;
sbit LCD_RW = P2^6;
sbit LCD_RS = P2^5;
sbit TLED = P2^4;
sbit Uout = P3^7;
sbit Bell = P2^0;
sfr LCD_DOUT = 0x80;
sfr key_interface = 0x90;
/******************************************
** 全局变量
******************************************/
K_MSG kmsg;
bit DisplayUpdataFlag,ClICk_Flag, BellingFlag, MeasureFlag, DelayFlag, MeaDelayExt;
uint8 T0_IRQ_Times, ShowPage, OBScure, PulseCoun, MeaDelayTime;
uint8 DisplayBuff[33];
uint8 RevTimeH;
uint16 RevTimeL;
uint32 RevTime;
uint8 code TestString[]={"www.huayimcu.com"};
/******************************************************************************/
/*******************************************************************************
** 函数名称: LcdDelay
** 功能描述: LCD控制时序用到的延时程序
**
** 输 入: times-延时量
**
** 输 出: 无
**
** 全局变量: 无
** 调用模块: 无
**-------------------------------------------------------------------------------
*********************************************************************************/
void LcdDelay(uint8 times)
{
while(times--);
}
/*******************************************************************************
** 函数名称: ReadLCDCR
** 功能描述: 读LCD命令寄存器
**
** 输 入: 无
**
** 输 出: (uint8) 读到的数据
**
** 全局变量: 无
** 调用模块: LcdDelay
*********************************************************************************/
uint8 ReadLCDCR(void)
{
uint8 byte;
LCD_RS = 0;
LCD_RW = 1;
LCD_E = 1;
LcdDelay(4);
byte = LCD_DOUT;
LCD_E = 0;
return(byte);
}
/*******************************************************************************
** 函数名称: WriteLCDCR
** 功能描述: 写LCD命令寄存器
**
** 输 入: (uint8 thdata) 将要写进寄存器的数据
**
** 输 出: 无
**
** 全局变量: 无
** 调用模块: LcdDelay
*********************************************************************************/
void WriteLCDCR(uint8 thEDAta)
{
LCD_DOUT = thedata;
LCD_RS = 0;
LCD_RW = 0;
LCD_E = 1;
LcdDelay(4);
LCD_E=0;
}
/*******************************************************************************
** 函数名称: WriteLCDDR
** 功能描述: 写LCD数据寄存器
**
** 输 入: (uint8 thedata) 将要写进寄存器的数据
**
** 输 出: 无
**
** 全局变量: 无
** 调用模块: LcdDelay
*********************************************************************************/
void WriteLCDDR(uint8 thedata)
{
LCD_DOUT = thedata;
LCD_RS = 1;
LCD_RW = 0;
LCD_E = 1;
LcdDelay(4);
LCD_E = 0;
}
/*******************************************************************************
** 函数名称: Test_LCDBF
** 功能描述: 检测LCD忙标志
**
** 输 入: 无
**
** 输 出: 无
**
** 全局变量: 无
** 调用模块: ReadLCDCR
**
*********************************************************************************/
void Test_LCDBF(void)
{
uint8 temp,ErrorTime;
ErrorTime = 0xff;
do
{
temp = ReadLCDCR();
ErrorTime--;
if(ErrorTime == 0)
break;
}
while(temp & 0x80);
}
/*******************************************************************************
** 函数名称: LCDInit
** 功能描述: LCD初始化
**
** 输 入: 无
**
** 输 出: 无
**
** 全局变量: 无
** 调用模块: WriteLCDCR
*********************************************************************************/
void LCDInit(void)
{
uint8 code custom_character[8][8] =
{/*自定义5X8字符*/
0x1f,0x00,0x00,0x00,0x00,0x00,0x00,0x00,/*自定义字符1*/
0x00,0x1f,0x00,0x00,0x00,0x00,0x00,0x00,/*自定义字符2*/
0x00,0x00,0x1f,0x00,0x00,0x00,0x00,0x00,/*自定义字符3*/
0x00,0x00,0x00,0x1f,0x00,0x00,0x00,0x00,/*自定义字符4*/
0x00,0x00,0x00,0x00,0x1f,0x00,0x00,0x00,/*自定义字符5*/
0x00,0x00,0x00,0x00,0x00,0x1f,0x00,0x00,/*自定义字符6*/
0x00,0x00,0x00,0x00,0x00,0x00,0x1f,0x00,/*自定义字符7*/
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x1f /*自定义字符8*/
};
uint8 i,j;
Test_LCDBF();
WriteLCDCR(0x38);
for(i=0x20; i>0; i--);
for(j=0xff; j>0; j--);
Test_LCDBF();
WriteLCDCR(0x38);
for(i=0x20; i>0; i--);
for(j=0xff; j>0; j--);
Test_LCDBF();
WriteLCDCR(0x38);
for(i=0x20; i>0; i--);
for(j=0xff; j>0; j--);
Test_LCDBF();
WriteLCDCR(0x06);
Test_LCDBF();
WriteLCDCR(0x0c);
Test_LCDBF();
WriteLCDCR(0x01);
Test_LCDBF();
WriteLCDCR(0x40);
for(i=0; i<=7; i++)
{
for(j=0; j<=7; j++)
{
Test_LCDBF();
WriteLCDDR(custom_character[i][j]);
}
}
}
/*******************************************************************************
** 函数名称: LCDDisplay
** 功能描述: LCD上显示字符串
**
** 输 入: *string(指向字符串地址),position(显示位置)
**
** 输 出: 无
**
** 全局变量: 无
** 调用模块: WriteLCDCR,WriteLCDDR,Test_LCDBF
*********************************************************************************/
void LCDDisplay(uint8 *string,uint8 position)
{
Test_LCDBF();
WriteLCDCR(position);
while(*string != ’#’)
{
if(position == 0x90)
{
Test_LCDBF();
WriteLCDCR(0xC0);
position = 0xC0;
}
/*if(*string == ’\0’)
*string = ’ ’; */
Test_LCDBF();
WriteLCDDR(*string++);
position++;
if(position > 0xCF)
{
Test_LCDBF();
WriteLCDCR(0x80);
position = 0x80;
}
}
}
/*******************************************************************************
** 函数名称: Timer0_IRQ
** 功能描述: 定时器0中断服务程序
**
** 输 入: 无
**
** 输 出: 无
**
** 全局变量:
** 调用模块: 无
**
*********************************************************************************/
void Timer0_IRQ(void) interrupt 1 using 1
{
TH0 = 0x3c;
TL0 = 0xa8;
if(++T0_IRQ_Times > 20)
{
T0_IRQ_Times = 0;
Click_Flag = ~Click_Flag;
//DisplayUpdataFlag = 1;
}
if(DelayFlag)
{
if(++MeaDelayTime > 10)
{
MeaDelayTime = 0;
DelayFlag = 0;
MeaDelayExt = 1;
}
}
}
/*******************************************************************************
** 函数名称: Timer0_IRQ
** 功能描述: 定时器1中断服务程序
**
** 输 入: 无
**
** 输 出: 无
**
** 全局变量:
** 调用模块: 无
**
*********************************************************************************/
void Timer1_IRQ(void) interrupt 3 using 2
{
RevTimeH++;
}
void IRQ_int0() interrupt 0 using 2 //INT1中断服务程序,使用第二组寄存器
{
EX0 = 0;
TR1 = 0;
RevTimeL = 256 * TH1 + TL1;
RevTime = RevTimeH * 65536 + RevTimeL;
if(RevTime < 1000)
{
BellingFlag = 1;
}
else
{
BellingFlag = 0;
}
DisplayUpdataFlag = 1;
TH1 = 0;
TL1 = 0;
}
/*******************************************************************************
** 函数名称: Updata_AD9850
** 功能描述: 更新AD9850(更新频率、相位数据)
**
** 输 入: 无
**
** 输 出: 无
**
** 全局变量:
** 调用模块: 无
*********************************************************************************/
void UpdataLCD(void)
{
uint8 temp8;
uint32 teMP32;
temp32 = RevTime;
DisplayBuff[16] = temp32 / 100000 + 48;
temp32 %= 100000;
DisplayBuff[17] = temp32 / 10000 + 48;
temp32 %= 10000;
DisplayBuff[18] = temp32 / 1000 + 48;
temp32 %= 1000;
DisplayBuff[19] = temp32 / 100 + 48;
temp32 %= 100;
DisplayBuff[20] = temp32 / 10 + 48;
temp8 = temp32 % 10;
DisplayBuff[21] = temp8 + 48;
LCDDisplay(DisplayBuff,0x80);
}
/*******************************************************************************
** 函数名称: main
** 功能描述: 主函数
**
** 输 入: 无
** 输 出: 无
**
** 全局变量:
** 调用模块: 无
*********************************************************************************/
void main(void)
{
//uint8 i;
TMOD = 0x11;
EA = 1;
ET0 = 1;
ET1 = 1;
IT0 = 1;
TR0 = 1;
LCDInit();
strcpy(DisplayBuff,TestString);
DisplayBuff[32] = ’#’;
DisplayUpdataFlag = 1;
MeaDelayExt = 1;
while(1)
{
TLED = Click_Flag;
if(DisplayUpdataFlag)
{
DisplayUpdataFlag = 0;
UpdataLCD();
MeasureFlag = 1;
}
if(MeasureFlag && MeaDelayExt)
{
RevTimeH = 0;
RevTimeL = 0;
RevTime = 0;
IE0 = 0;
TF1 = 0;
EX0 = 1;
TR1 = 1;
for(PulseCoun = 0; PulseCoun<10; PulseCoun++)
{
Uout = ~Uout;
delayus();
}
MeasureFlag = 0;
MeaDelayExt = 0;
DelayFlag = 1;
}
if(BellingFlag)
{
Bell = 0;
}
else
{
Bell = 1;
}
}
}
上一篇:三相步进电机驱动电路
下一篇:智能家居控制系统C程序
推荐阅读最新更新时间:2024-03-16 15:52
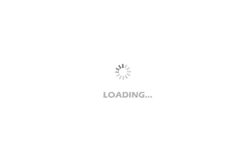
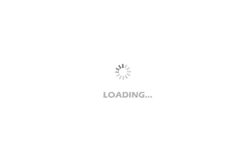
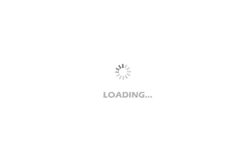
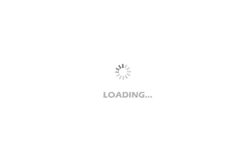