#pragma small
#include
#include
/********************************************
* DS1302 PIN Configuration *
********************************************
sbit DS_CLK = P1^6
sbit DS_IO = P1^5;
sbit DS_RST = P1^4;
/********************************************
* Shift Data from Mcu in DS1302 *
********************************************/
void DS_Shift_In(unsigned char bIn)
{
unsigned char i;
for( i=0;i<8;i++ )
{
DS_CLK = 0; /* low logIC level */
if( bIn&0x01 ) DS_IO = 1; /* change data */
else DS_IO = 0; /* LSB first */
bIn = bIn>>1; /* next bit */
DS_CLK = 1; /* raising edge in */
_nop_(); /* delay */
_nop_();
}
}
/********************************************
* Shift Data from DS1302 to MCU *
********************************************/
unsigned char DS_Shift_Out(void)
{
unsigned char i, bData;
DS_IO=1; /* release DS_IO */
for( i=0;i<8;i++ )
{
DS_CLK = 1; /* high logic level */
_nop_(); /* delay */
_nop_();
DS_CLK = 0; /* failing edge data removed */
_nop_(); /* delay */
_nop_();
bData = bData>>1; /* store data */
if( DS_IO ) bData = bData|0x80 ;
}
return (bData);
}
/********************************************
* Read One Byte from DS1302 to MCU *
* Written in 25/10,2000. HIT *
* cr: Read Command *
* 0x81-Read Second: CH XXX,XXXX(BCD) *
* 0X83-Read Minute: 0 XXX,XXXX(BCD) *
* 0X85-Read Hour: 12/24 0 A/P x,xxxx *
* 0X87-Read Date: 00 XX,XXXX(BCD) *
* 0X89-Read Month: 000 X,XXXX(BCD) *
* 0X8B-Read Week: 00000 XXX(BCD) *
* 0X8D-Read Year: xxxx XXX(BCD) *
********************************************/
unsigned char DS_Read( unsigned char cr )
{
unsigned char dd=0;
DS_RST = 0; /* initializing */
_nop_();
DS_CLK = 0; /* SCLK low logic level */
_nop_();
DS_RST = 1; /* EnabLED */
DS_Shift_In( cr ); /* Write Command Byte */
dd = DS_Shift_Out(); /* Read Data */
DS_RST=0; /* DISAbled */
DS_CLK=1;
return ( dd );
}
/*********************************************
* Read One Byte from DS1302 to MCU *
* Written in 25/10,2000. HIT *
* ord: Write Command,dd-data for writting*
* 0x80-Write Second: CH XXX,XXXX(BCD) *
* 0X82-Write Minute: 0 XXX,XXXX(BCD) *
* 0X84-Write Hour: 12/24 0 A/P x,xxxx *
* 0X85-Write Date: 00 XX,XXXX(BCD) *
* 0X86-Write Month: 000 X,XXXX(BCD) *
* 0X8a-Write Week: 00000 XXX(BCD) *
* 0X8c-Write Year: xxxx XXX(BCD) *
*********************************************/
void DS_Write(unsigned char ord,unsigned char dd)
{
DS_RST = 0;
_nop_();
DS_CLK = 0;
_nop_();
DS_RST = 1; /* Enable */
DS_Shift_In( ord ); /* Write Command */
DS_Shift_In( dd ); /* Write Data */
DS_RST = 0;
DS_CLK = 1;
}
/*********************************************
* Set DS1302 CLOCk Value *
* Written in 25/10,2000. HIT *
* dt[6]-dt[0]: Week,Year,Month,Date *
* Hour,Minute,Second *
*********************************************/
void DS_SetClock(unsigned char dt[7])
{
DS_Write(0x8E,0); /* Disabled Write Protect */
DS_Write(0x80,0x80); /* Disabled Clock */
DS_Write(0x8a,dt[6]); /* Week Day BCD */
DS_Write(0x8c,dt[5]); /* Year : BCD */
DS_Write(0x88,dt[4]); /* Month : BCD */
DS_Write(0x86,dt[3]); /* Date : BCD */
DS_Write(0x84,dt[2]); /* Hour(24) BCD */
DS_Write(0x82,dt[1]); /* Minute : BCD */
DS_Write(0x80,dt[0]&0x7F); /* Second : BCD */
/* MSB:0 Enabled Clock */
DS_Write(0x8E,0x80); /* Enabled Write Protect */
}
/*********************************************
* Read DS1302 Clock Value *
* Written in 25/10,2000. HIT *
* dt[6]-dt[0]: Week,Year,Month,Date *
* Hour,Minute,Second *
*********************************************/
void DS_ReadClock(unsigned char dt[7])
{
dt[6]=DS_Read(0x8b); /* Week */
dt[5]=DS_Read(0x8d); /* Year */
dt[4]=DS_Read(0x89); /* Month */
dt[3]=DS_Read(0x87); /* Date */
dt[2]=DS_Read(0x85)&0x3F; /* Hour */
dt[1]=DS_Read(0x83)&0x7F; /* Minute */
dt[0]=DS_Read(0x81)&0x7F; /* Second */
}
上一篇:实用单片机电子钟的制作
下一篇:51读写串行EEPROM芯片at2402程序(C)
推荐阅读最新更新时间:2024-03-16 15:53
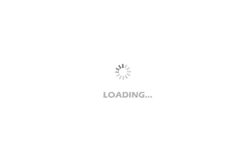
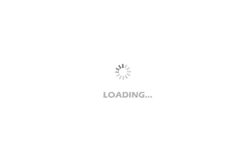
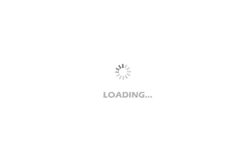