1、使用2440 iic 模块控制
#include "2440addr.h"
#include "uart.h"
volatile int ackFlag = 0;
void delay()
{
int i,j;
for(i = 0; i < 500;i++)
for(j = 0; j < 500; j++)
;
}
/***********************************************
Function name : Iic_ISR
Description : iic 中断处理函数
Input parameter : none
Return : none
Others :
*************************************************/
static void __irq Iic_ISR()
{
//清除中断
rSRCPND |= (1 << 27);
rINTPND |= (1 << 27);
ackFlag = 1;
}
/***********************************************
Function name : iic_init
Description : iic 通信初始化
Input parameter : none
Return : none
Others :
*************************************************/
void iic_init(void)
{
//配置GPE1415 为 IICSCL 、IICSDA、不使能拉高电阻(GPE1415没有对应的位)
rGPECON &= ~(0xf << 28);
rGPECON |= (0x2 << 30) | (0x2 << 28);
//清除中断
rSRCPND |= (1 << 27);
rINTPND |= (1 << 27);
//开启iic中断
rINTMSK &= ~(1 << 27);
//设置中断处理函数
pISR_IIC = (unsigned)Iic_ISR;
//配置IICCON寄存器:使能ACK,时钟源选择fPCLK /512
//(从eeprom手册看出,其最大的传输频率为100kHZ)
//使能中断,发送时钟值为0xf。
//Tx clock = IICCLK/(IICCON[3:0]+1)=50000000/512=97kHz
rIICCON = (1<<7)|(1<<6)|(1<<5);
//设置CPU的从设备地址(未使用)
rIICADD = 0x10;
//IIC-bus data output enable
rIICSTAT |= (1<<4);
}
/***********************************************
Function name : iic_Tx
Description : iic 发送数据
Input parameter : buffer 要发送的数据
size:数据大小
Return : none
Others :
*************************************************/
void iic_Tx(unsigned char devAddr,unsigned char wordAddr,char buffer[],int size)
{
int i = 0,j = 0;
ackFlag = 0;
//写从设备地址到IICDS寄存器
rIICDS = devAddr;
//配置为主发送模式
//写0XF0到IICSTAT(主发送模式,并开始)
rIICSTAT = 0xf0;
for(i = 0; i < 100; i++);
//清除挂起位
rIICCON &= ~(1<<4);
//等待ack中断响应
//while(!ackFlag);
//注意:这里等待挂起可以单独判断pending,但不能单独判断ack,防止数据丢失
while(!ackFlag)
delay();
ackFlag = 0;
//写起始地址到IICDS寄存器
rIICDS = wordAddr;
for(i = 0; i < 100; i++);
//清除挂起位
rIICCON &= ~(1<<4);
//等待ack中断响应
while(!ackFlag)
delay();
ackFlag = 0;
//向IICDS写入发送数据直到发送结束
for(j = 0; j < size; j++)
{
//写入新数据
rIICDS = buffer[j];
for(i = 0; i < 100; i++);
//清除挂起位
rIICCON &= ~(1<<4);
//等待ack中断
while(!ackFlag)
delay();
ackFlag = 0;
}
//发送结束,写0XD0到 IICSTAT
rIICSTAT = 0xd0;
rIICCON = 0xe0;
//等待停止条件生效
delay();
}
/***********************************************
Function name : iic_Rx
Description : iic 接收数据
Input parameter : buffer 接收buffer
Return : i: 接收到的数据大小
Others :
*************************************************/
int iic_Rx(unsigned char devAddr,unsigned char wordAddr,char * buffer,int size)
{
char ch;
int i = 0,j = 0;
ackFlag = 0;
//写从设备地址到IICDS寄存器
rIICDS = devAddr;
for(i = 0; i < 100; i++);
//配置为主发送模式
//写0XF0到IICSTAT(主发送模式,并开始)
rIICSTAT = 0xf0;
//清除挂起位
rIICCON &= ~0x10;
//等待ack中断响应
while(!ackFlag)
delay();
ackFlag = 0;
//写起始地址到IICDS寄存器
rIICDS = wordAddr;
for(i = 0; i < 100; i++);
//清除挂起位
rIICCON &= ~0x10;
//等待ack中断响应
while(!ackFlag)
delay();
ackFlag = 0;
//写从设备地址到IICDS寄存器
rIICDS = devAddr;
for(i = 0; i < 100; i++);
//写0XB0到IICSTAT(主接收模式,并开始)
rIICSTAT = 0xb0;
//清除挂起位
rIICCON &= ~0x10;
//等待ack中断响应
while(!ackFlag)
delay();
ackFlag = 0;
//从IICDS读取第一个字节舍弃
ch = rIICDS;
//清除挂起位
rIICCON &= ~0x10;
//等待ack中断响应
while(!ackFlag)
delay();
ackFlag = 0;
//从IICDS读取数据直到接收结束
for(j = 0; j < size; j++)
{
if(j == size-1)
{
rIICCON &= ~0x80;
}
//从IICDS读取数据
buffer[j] = rIICDS;
for(i=0;i<100;i++);
//清除挂起位
rIICCON &= ~0x10;
//等待ack中断响应
while(!ackFlag)
delay();
ackFlag = 0;
}
//接收结束,写0X90到 IICSTAT
rIICSTAT = 0x90;
rIICCON = 0xe0;
//等待停止条件生效
delay();
return j;
}
/***********************************************
Function name : iic_test
Description : iic 测试
Input parameter : none
Return : none
Others :
*************************************************/
int iic_test(void)
{
iic_init();
int i = 998;
int j = 0;
iic_Tx(0xa0,0,(char*)&i,4);
iic_Rx(0xa0,0,(char*)&j,4);
uart_printf("nj = %dn",j);
}
2、使用 io 模拟
/******************
iic.h
*******************/
#ifndef _IIC_H_
#define _IIC_H_
#include "def.h"
void iic_init(void);
#define AT24C08_ADDRDRESS 0xa0
#define true 1
#define false 0
#define PAGE1 0xa0
#define PAGE2 0xa2
#define PAGE3 0xa4
#define PAGE4 0xa6
#define IIC_CLOCK_HIGH rGPEDAT |= (1<<14)
#define IIC_CLOCK_LOW rGPEDAT &= ~(1<<14)
#define IIC_DATA_HIGH rGPEDAT |= (1<<15)
#define IIC_DATA_LOW rGPEDAT &= ~(1<<15)
#define SET_IIC_DATA_INPUT rGPECON &= ~(3<<30)
#define SET_IIC_DATA_OUTPUT rGPECON |= (1<<30)
U8 i2c_write(U8 device_address, U8 memory_address , U8 data);
U8 i2c_read(U8 device_address, U8 memory_address, U8 *data);
U8 i2c_page_read(U8 device_address, U8 memory_address, U8 *destination,U32 size);
U8 i2c_page_write(U8 device_address, U8 memory_address , U8 *source,U32 size);
U8 i2c_largepage_write(U8 device_address, U8 memory_address , U8 *source,U32 size);
void i2c_test(void);
#endif
/*****************************
iic.c
*****************************/
#include "2440addr.h"
#include "def.h"
#include "uart.h"
#include "iic.h"
//#include "timer.h"
#define DELAY_DECIMUS_MS 100
extern void Delay_MS( unsigned int time);
void delayus( U32 time)
{
U32 i,j;
for ( i=0; i
上一篇:s3c2440中断总结+按键中断
下一篇:s3c2440串口编程
推荐阅读最新更新时间:2024-11-18 16:29
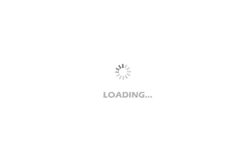
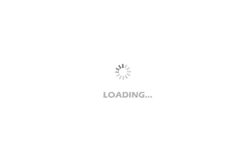
设计资源 培训 开发板 精华推荐
- 适用于汽车应用的 A5970D 1A 降压稳压器的典型应用板
- DC2085A-F,具有 LTC2000A-11、11 位、2.7Gsps DAC 和 DDR LVDS 接口的演示板
- AM2G-1205SH30Z 5V 2W DC-DC 转换器的典型应用
- NCP1343PD100WGEVB:NCP1343 100 W USB PD 评估板
- 白嫖信仰尺!
- 使用 California Eastern Laboratories 的 THCV218 的参考设计
- Typical Application Circuit for LTC3526EDC 3.3V Boost Converter with Output OR'd with 5V USB Input
- 使用 Cypress Semiconductor 的 CY8C21634 的参考设计
- 96W、12V DC 至 DC 单路输出电源,用于适配器 AC 至 DC 电源
- homework1